Understanding and Implementing Regex for Email Verification
In today’s post, we’re going to dive into a complex yet fascinating topic – Regular Expressions, or Regex, specifically for email verification. This is a powerful tool that anyone dealing with text processing should have in their toolkit.
What is Regex?
Regular Expressions (Regex) are a powerful way to analyze text. They allow you to create search patterns that can match, locate, and manage text. Regex can be as simple or as complex as you make it. It’s a versatile tool used across various fields and applications such as search engines, text editors, programming languages, and more.
Why Do We Need Regex for Email Verification?
Imagine you’re developing an application, and you need to ensure that users provide valid email addresses during registration. Writing a function to check every possible condition can be tedious. This is where Regex comes into play. You can write a regex pattern that adheres to the standard format of an email address, thus simplifying the process of validating email addresses.
Regex for Email Verification
Let’s go over a regex pattern for email verification.
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
Let’s break down this pattern to understand how it works:
^
: This symbol is used to start of the line.[a-zA-Z0-9._%+-]+
: This ensures that the email starts with one or more of any of the allowed characters (a-z, A-Z, 0-9, ., _, %, +, and -).@
: This matches the @ symbol. An email address must have one and only one @ symbol.[a-zA-Z0-9.-]+
: This matches the domain name which can have one or more of any of the allowed characters (a-z, A-Z, 0-9, ., and -).\.
: This matches the period (.). Note the backslash, which is an escape character because a period is a special character in regex. An email address must have a period in the domain name.[a-zA-Z]{2,}
: This matches the domain suffix, such as .com, .net, .org, etc. It must have at least 2 letters (a-z or A-Z).$
: This symbol is used to denote the end of the line.
This regular expression can handle many common forms of email addresses. However, it isn’t perfect. It doesn’t handle all possibilities defined in the RFC 5322 Standard which defines the email formats. Implementing a fully compliant regex pattern can be significantly more complicated due to the breadth of potential formats allowed.
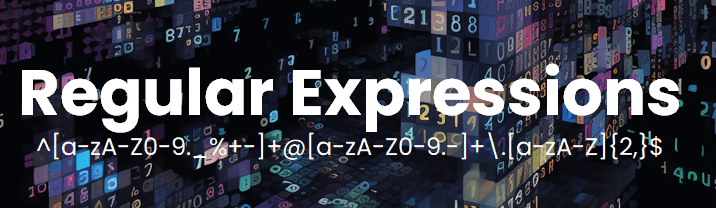
Implementing Regex in Different Programming Languages
Most modern programming languages support Regex, and implementation is often very straightforward. Here’s how you can use the above regex pattern to validate email addresses in a few popular languages:
JavaScript:
function validateEmail(email) {
var regex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
return regex.test(email);
}
Python:
import re
def validate_email(email):
regex = '^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
if re.match(regex, email):
return True
else:
return False
Java:
import java.util.regex.*;
public class Main {
public static void main(String[] args) {
String email = "example@example.com";
String regex = "^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,}$";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(email);
System.out.println(matcher.matches());
}
}
PHP
function validateEmail($email) {
$regex = "/^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/";
if (preg_match($regex, $email)) {
return true;
} else {
return false;
}
}
You can use this function in your PHP code like this:
$email = "example@example.com";
if(validateEmail($email)) {
echo "Email is valid.";
} else {
echo "Email is not valid.";
}
This script will print “Email is valid.” if the email address fits the pattern described in the regex and “Email is not valid.” if it does not. Remember that while this regex pattern is quite thorough, it doesn’t cover all possible valid email address formats as per the RFC 5322 Standard. You should consider this function as the first step in the email verification process and use other methods like sending a confirmation email for complete verification.
RFC Standards
The structure and standards of email addresses have been outlined in a series of Request for Comments (RFC) documents. The most significant among these are RFC 822, RFC 2822, and RFC 5322.
1. RFC 822 – Standard for ARPA Internet Text Messages
Published in August 1982, RFC 822 was the first standard to define the format of email messages. It also defined the basic structure of email addresses as local-part@domain
. This structure is still widely in use today.
2. RFC 2822 – Internet Message Format
This standard, which superseded RFC 822, was published in April 2001. It expanded and clarified many of the rules. According to RFC 2822, the local part could be up to 64 characters long and the domain name could be up to 255 characters long. The local part may use any of these ASCII characters:
- Uppercase and lowercase English letters (a–z, A–Z)
- Digits 0 to 9
- Characters ! # $ % & ‘ * + – / = ? ^ _ ` { | } ~
- Dot ., provided that it is not the first or last character, and provided also that it does not appear two or more times consecutively.
The domain name part of an email address has to conform to strict guidelines: it must match the requirements for a hostname, consisting of letters, digits, hyphens, and dots.
3. RFC 5322 – Internet Message Format
Published in October 2008, RFC 5322 superseded RFC 2822. It maintained the same overall structure of the email address and brought in more specifics and modern rules, taking into account the internationalization of email addresses (see RFC 6531).
For example, although the standard does not limit the character set in the local part to ASCII characters, servers are encouraged to adopt UTF-8 as a superset of ASCII and to accommodate the user-friendly quoted-string syntax and the dot-atom syntax for the local part. An example of such a complex email address would be "very.(),:;<>[]\".VERY.\"very@\\ \"very\".unusual"@strange.example.com
.
4. RFC 6531 – SMTP Extension for Internationalized Email
Published in February 2012, RFC 6531 allows non-ASCII characters to be used in both the local parts and domain of an email address. RFC 6531 provides a specification for how these internationalized email addresses should be processed. Allowing UTF-8 encoding within the message header and in the addresses was a significant step towards the internationalization of the email.
The flexibility and variety of email address formats are a result of decades of technological evolution closely tied to the push-and-pull of technology and culture. However, the standards described above are not always adhered to. Some systems are more permissive, allowing space or other non-ASCII or control characters, while others, like the widely used post-verification system, are more restrictive.
Therefore, the best practices for validating an email address are not only to check its syntax with a regular expression but also to verify the existence of the email domain and to send a confirmation email to the address to ensure its accuracy and ownership.
Regex is a powerful tool when it comes to dealing with text pattern searching and manipulation tasks, such as email verification. Although the example regex we provided isn’t comprehensive, it gives a good starting point for the most common formats.
When using regex for email validation, it’s crucial to remember that no matter how complicated your regex pattern is, it might still fail to cover all possibilities. Thus, it should never be the only means of verification. It’s always recommended to use other email verification methods, like sending a confirmation email to the provided address.
We hope you found this post helpful and that it has deepened your understanding of regular expressions and their application to email verification. Stay tuned for more insightful discussions on software development topics!